[React+Tailwind] Toast を TypeScript に書き直してみた | 心を無にして始める React
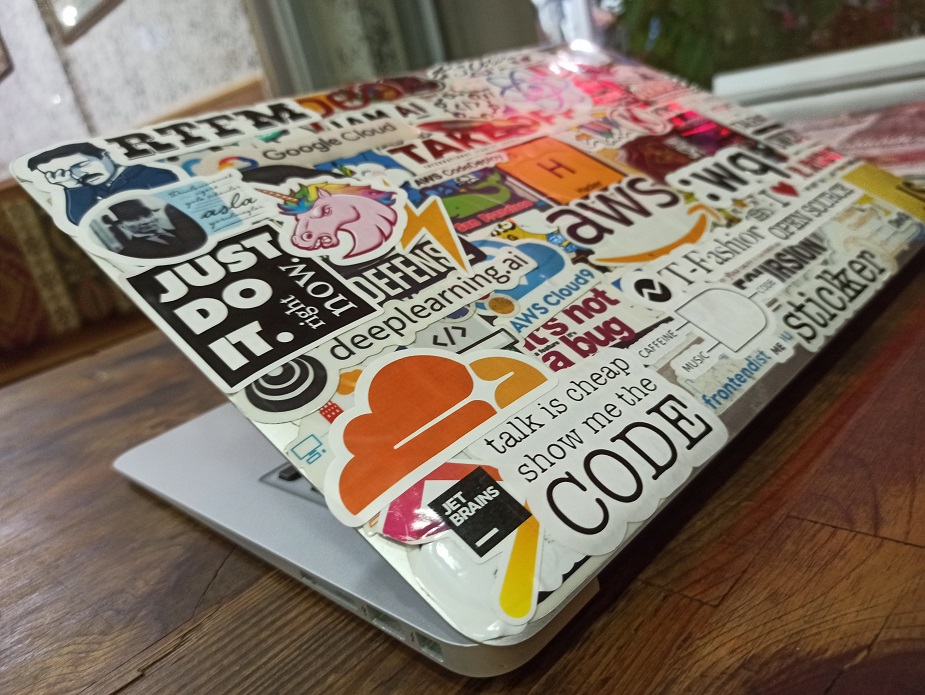
準備
書き直すベースはこちらの Toast コンポーネントです。
JavaScript
ベースの Toast.js です。
import React, { forwardRef } from 'react';
import {
Toast as FlowbiteToast,
} from 'flowbite-react';
const Toast = forwardRef((
{
children,
duration,
...otherProps
},
ref,
) => {
return (
<FlowbiteToast
duration={duration}
{...otherProps}
>
{children}
</FlowbiteToast>
);
});
const ToastToggle = forwardRef((
{
children,
xIcon,
...otherProps
},
ref,
) => {
return (
<FlowbiteToast.Toggle
xIcon={xIcon}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteToast.Toggle>
);
});
export default Object.assign(Toast, {
Toggle: ToastToggle,
});
TypeScript
Toast.tsx として編集します。
import React, { ComponentProps, forwardRef, LegacyRef } from 'react';
import {
Toast as FlowbiteToast,
ToastProps as FlowbiteToastProps,
} from 'flowbite-react';
type ToastProps = {} & FlowbiteToastProps;
const Toast = forwardRef((
{
children,
duration,
...otherProps
}: ToastProps,
ref: LegacyRef<HTMLDivElement>,
) => {
return (
<FlowbiteToast
duration={duration}
{...otherProps}
>
{children}
</FlowbiteToast>
);
});
type ToastToggleProps = {} & ComponentProps<typeof FlowbiteToast.Toggle>;
const ToastToggle = forwardRef((
{
children,
xIcon,
...otherProps
}: ToastToggleProps,
ref: LegacyRef<HTMLButtonElement>,
) => {
return (
<FlowbiteToast.Toggle
xIcon={xIcon}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteToast.Toggle>
);
});
export default Object.assign(Toast, {
Toggle: ToastToggle,
});
結果
変化はないので、前と同じ。
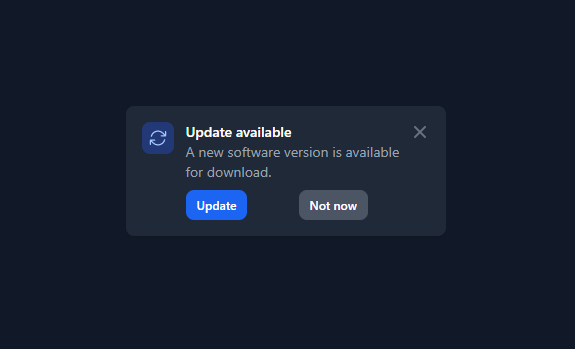
はい、できました。