[React+Tailwind] Tab を TypeScript に書き直してみた | 心を無にして始める React
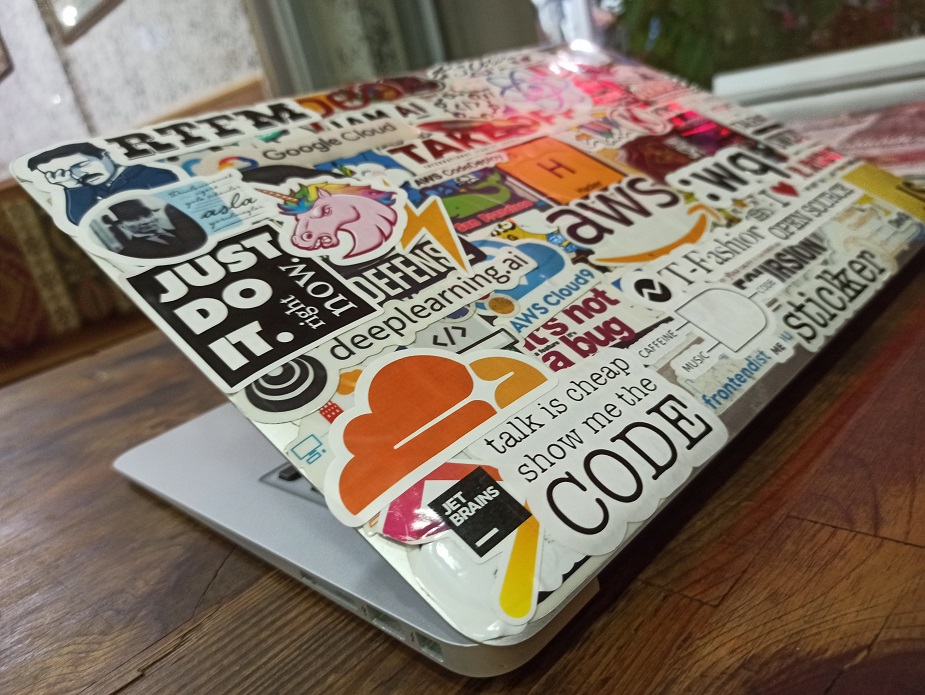
準備
書き直すベースはこちらの Tabs コンポーネントです。
JavaScript
ベースの Tabs.js です。
import React, { forwardRef } from 'react';
import {
Tabs as FlowbiteTabs,
} from 'flowbite-react';
const TabsGroup = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTabs.Group
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTabs.Group>
);
});
const TabsItem = forwardRef((
{
active = false,
children,
disabled = false,
icon,
title,
...otherProps
},
ref,
) => {
return (
<FlowbiteTabs.Item
active={active}
disabled={disabled}
icon={icon}
ref={ref}
title={title}
{...otherProps}
>
{children}
</FlowbiteTabs.Item>
);
});
const Tabs = {
Group: TabsGroup,
Item: TabsItem,
};
export default Tabs;
TypeScript
Tabs.tsx として編集します。
import React, { ComponentProps, forwardRef, LegacyRef } from 'react';
import {
Tabs as FlowbiteTabs,
} from 'flowbite-react';
type TabsGroupProps = {} & ComponentProps<typeof FlowbiteTabs.Group>;
const TabsGroup = forwardRef((
{
children,
...otherProps
}: TabsGroupProps,
ref: LegacyRef<HTMLDivElement>,
) => {
return (
<FlowbiteTabs.Group
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTabs.Group>
);
});
type TabsItemProps = {} & ComponentProps<typeof FlowbiteTabs.Item>;
const TabsItem = forwardRef((
{
active = false,
children,
disabled = false,
icon,
title,
...otherProps
}: TabsItemProps,
ref: LegacyRef<HTMLDivElement>,
) => {
return (
<FlowbiteTabs.Item
active={active}
disabled={disabled}
icon={icon}
ref={ref}
title={title}
{...otherProps}
>
{children}
</FlowbiteTabs.Item>
);
});
const Tabs = {
Group: TabsGroup,
Item: TabsItem,
};
export default Tabs;
結果
変化はないので、前と同じ。
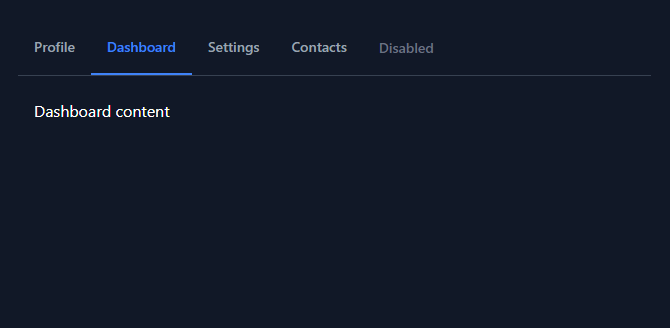
はい、できました。