[React+Tailwind] Navbar を TypeScript に書き直してみた | 心を無にして始める React
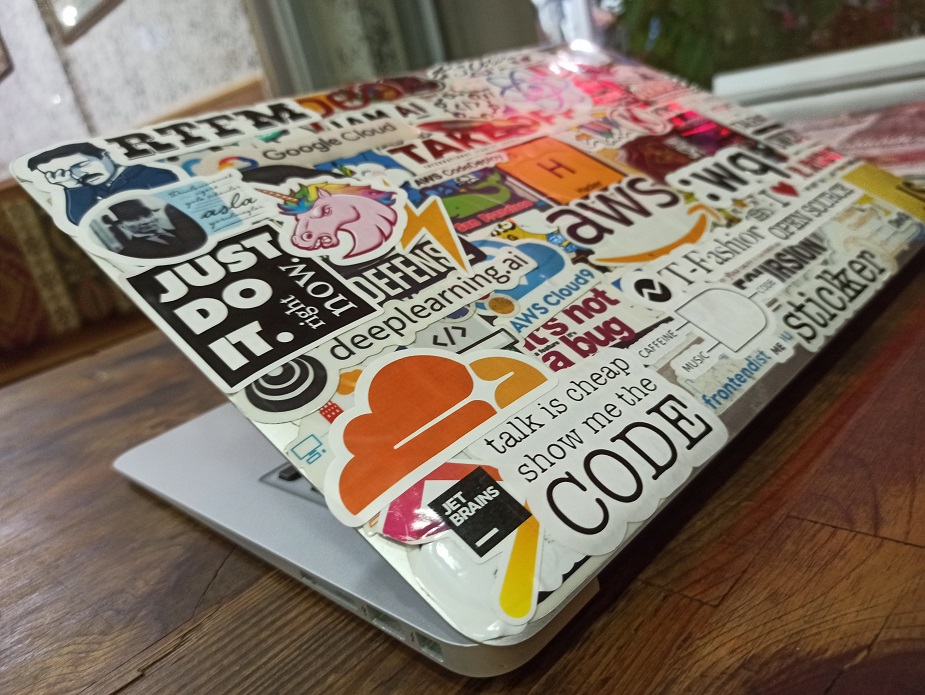
準備
書き直すベースはこちらの Navbar コンポーネントです。
JavaScript
ベースの Navbar.js です。
import React, { forwardRef } from 'react';
import {
Navbar as FlowbiteNavbar,
} from 'flowbite-react';
const Navbar = forwardRef((
{
border = false,
children,
fluid = true,
menuOpen = false,
rounded = false,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar
border={border}
fluid={fluid}
menuOpen={menuOpen}
ref={ref}
rounded={rounded}
{...otherProps}
>
{children}
</FlowbiteNavbar>
);
});
const NavbarBrand = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Brand
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Brand>
);
});
const NavbarCollapse = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Collapse
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Collapse>
);
});
const NavbarLink = forwardRef((
{
active = false,
children,
disabled = false,
href,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Link
active={active}
disabled={disabled}
href={href}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Link>
);
});
const NavbarToogle = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Toggle
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Toggle>
);
});
export default Object.assign(Navbar, {
Brand: NavbarBrand,
Collapse: NavbarCollapse,
Link: NavbarLink,
Toggle: NavbarToogle,
});
TypeScript
Navbar.tsx として編集します。
import React, { ComponentProps, forwardRef } from 'react';
import {
Navbar as FlowbiteNavbar,
NavbarComponentProps as FlowbiteNavbarProps,
} from 'flowbite-react';
import { LegacyRef } from 'react';
type NavbarProps = {} & FlowbiteNavbarProps;
const Navbar = forwardRef((
{
border = false,
children,
fluid = true,
menuOpen = false,
rounded = false,
...otherProps
}: NavbarProps,
ref: LegacyRef<HTMLDivElement>,
) => {
return (
<FlowbiteNavbar
border={border}
fluid={fluid}
menuOpen={menuOpen}
ref={ref}
rounded={rounded}
{...otherProps}
>
{children}
</FlowbiteNavbar>
);
});
type NavbarBrandProps = {} & ComponentProps<typeof FlowbiteNavbar.Brand>;
const NavbarBrand = forwardRef((
{
children,
...otherProps
}: NavbarBrandProps,
ref: LegacyRef<HTMLAnchorElement>,
) => {
return (
<FlowbiteNavbar.Brand
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Brand>
);
});
type NavbarCollapseProps = {} & ComponentProps<typeof FlowbiteNavbar.Collapse>;
const NavbarCollapse = forwardRef((
{
children,
...otherProps
}: NavbarCollapseProps,
ref: LegacyRef<HTMLDivElement>,
) => {
return (
<FlowbiteNavbar.Collapse
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Collapse>
);
});
type NavbarLinkProps = {} & ComponentProps<typeof FlowbiteNavbar.Link>;
const NavbarLink = forwardRef((
{
active = false,
children,
disabled = false,
href,
...otherProps
}: NavbarLinkProps,
ref: LegacyRef<HTMLAnchorElement>,
) => {
return (
<FlowbiteNavbar.Link
active={active}
disabled={disabled}
href={href}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Link>
);
});
type NavbarToggleProps = {} & ComponentProps<typeof FlowbiteNavbar.Toggle>;
const NavbarToogle = forwardRef((
{
children,
...otherProps
}: NavbarToggleProps,
ref: LegacyRef<HTMLButtonElement>,
) => {
return (
<FlowbiteNavbar.Toggle
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Toggle>
);
});
export default Object.assign(Navbar, {
Brand: NavbarBrand,
Collapse: NavbarCollapse,
Link: NavbarLink,
Toggle: NavbarToogle,
});
結果
変化はないので、前と同じ。
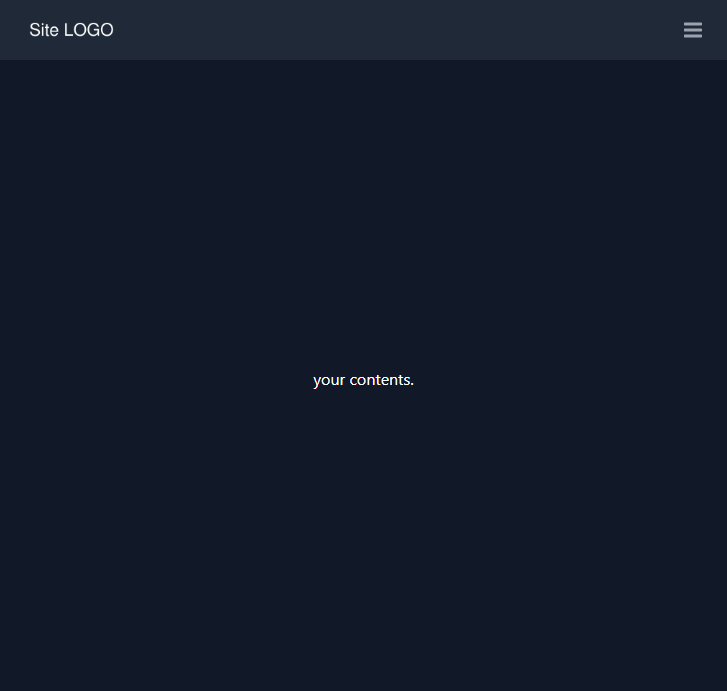
はい、できました。