[React+Tailwind] Rating を TypeScript に書き直してみた | 心を無にして始める React
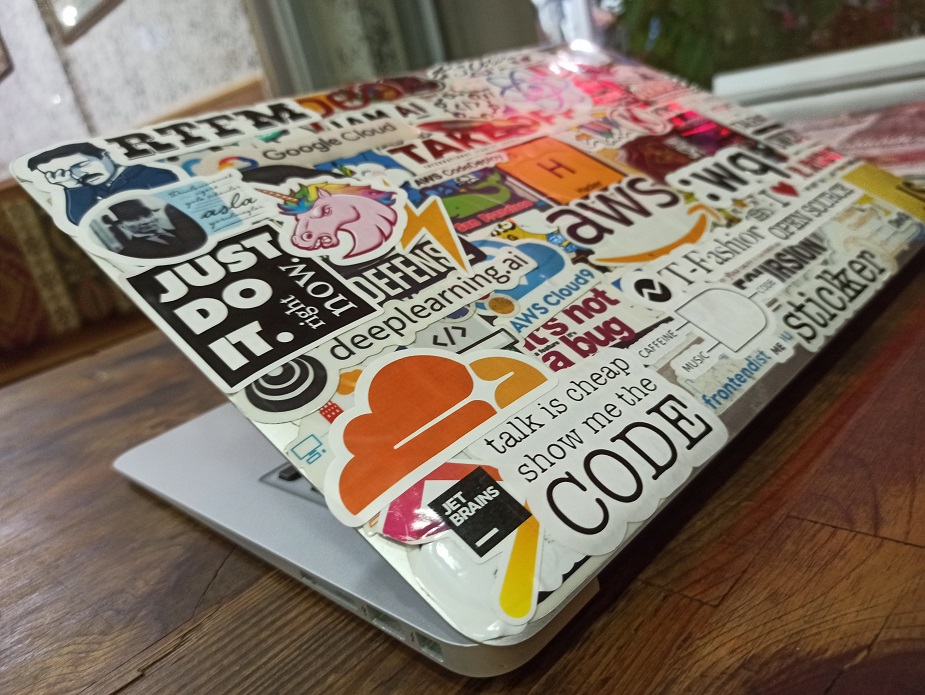
準備
書き直すベースはこちらの Rating コンポーネントです。
JavaScript
ベースの Rating.js です。
import React, { forwardRef } from 'react';
import {
Rating as FlowbiteRating,
} from 'flowbite-react';
const Rating = forwardRef((
{
children,
size,
...otherProps
},
ref,
) => {
return (
<FlowbiteRating
ref={ref}
size={size}
{...otherProps}
>
{children}
</FlowbiteRating>
);
});
const RatingStar = (
{
filled = false,
starIcon,
...otherProps
}
) => {
return (
<FlowbiteRating.Star
filled={filled}
starIcon={starIcon}
{...otherProps}
/>
);
};
const RatingAdvanced = forwardRef((
{
children,
percentFilled,
...otherProps
},
ref,
) => {
return (
<FlowbiteRating.Advanced
percentFilled={percentFilled}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteRating.Advanced>
);
});
export default Object.assign(Rating, {
Star: RatingStar,
Advanced: RatingAdvanced,
});
TypeScript
Rating.tsx として編集します。
import React, { ComponentProps, forwardRef } from 'react';
import {
Rating as FlowbiteRating,
RatingProps as FlowbiteRatingProps,
} from 'flowbite-react';
import { LegacyRef } from 'react';
type RatingProps = {} & FlowbiteRatingProps;
const Rating = forwardRef((
{
children,
size,
...otherProps
}: RatingProps,
ref: LegacyRef<HTMLDivElement>,
) => {
return (
<FlowbiteRating
ref={ref}
size={size}
{...otherProps}
>
{children}
</FlowbiteRating>
);
});
type RatingStarProps = {} & ComponentProps<typeof FlowbiteRating.Star>;
const RatingStar = (
{
filled = false,
starIcon,
...otherProps
}: RatingStarProps,
) => {
return (
<FlowbiteRating.Star
filled={filled}
starIcon={starIcon}
{...otherProps}
/>
);
};
type RatingAdvancedProps = {} & ComponentProps<typeof FlowbiteRating.Advanced>;
const RatingAdvanced = forwardRef((
{
children,
percentFilled,
...otherProps
}: RatingAdvancedProps,
ref: LegacyRef<HTMLDivElement>,
) => {
return (
<FlowbiteRating.Advanced
percentFilled={percentFilled}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteRating.Advanced>
);
});
export default Object.assign(Rating, {
Star: RatingStar,
Advanced: RatingAdvanced,
});
結果
変化はないので、前と同じ。
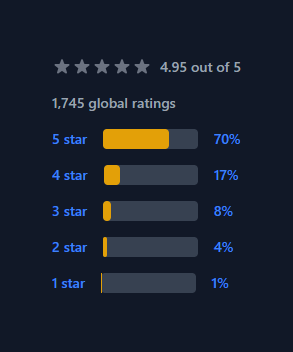
はい、できました。