[React+Tailwind] Flowbite で Timeline を表示してみる | 心を無にして始める React
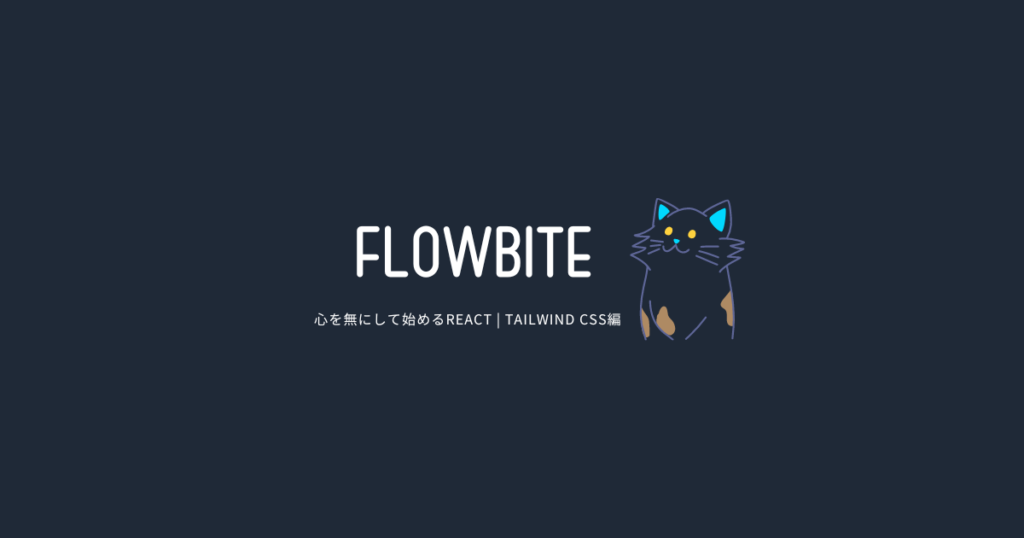
準備
Flowbite が使えるプロジェクトを準備します。
Timeline コンポーネントをつくる
./src/components 配下に Timeline.js を作ります。
import React, { forwardRef } from 'react';
import {
Timeline as FlowbiteTimeline,
} from 'flowbite-react';
const Timeline = (
{
children,
className,
horizontal,
...otherProps
},
) => {
return (
<FlowbiteTimeline
className={className}
horizontal={horizontal}
{...otherProps}
>
{children}
</FlowbiteTimeline>
);
};
const TimelineItem = forwardRef((
{
children,
className,
...otherProps
},
ref,
) => {
return (
<FlowbiteTimeline.Item
className={className}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTimeline.Item>
);
});
const TimelinePoint = forwardRef((
{
children,
className,
icon,
...otherProps
},
ref,
) => {
return (
<FlowbiteTimeline.Point
className={className}
icon={icon}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTimeline.Point>
);
});
const TimelineContent = forwardRef((
{
children,
className,
...otherProps
},
ref,
) => {
return (
<FlowbiteTimeline.Content
className={className}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTimeline.Content>
);
});
const TimelineTime = forwardRef((
{
children,
className,
...otherProps
},
ref,
) => {
return (
<FlowbiteTimeline.Time
className={className}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTimeline.Time>
);
});
const TimelineTitle = forwardRef((
{
as,
children,
className,
...otherProps
},
ref,
) => {
return (
<FlowbiteTimeline.Title
as={as}
className={className}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTimeline.Title>
);
});
const TimelineBody = forwardRef((
{
children,
className,
...otherProps
},
ref,
) => {
return (
<FlowbiteTimeline.Body
className={className}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTimeline.Body>
);
});
export default Object.assign(Timeline, {
Item: TimelineItem,
Point: TimelinePoint,
Content: TimelineContent,
Time: TimelineTime,
Title: TimelineTitle,
Body: TimelineBody,
});
Timeline コンポーネントをつかう
いつものように App.js を編集していきます。
import { ArrowSmallRightIcon } from '@heroicons/react/24/solid';
import './App.css';
import Timeline from './components/Timeline';
import Button from './components/Button';
function App() {
return (
<div className="min-h-screen w-full gap-4 flex flex-col justify-center items-center dark:text-white dark:!bg-gray-900">
<div className="w-full p-8">
<Timeline>
<Timeline.Item>
<Timeline.Point />
<Timeline.Content>
<Timeline.Time>
February 2022
</Timeline.Time>
<Timeline.Title>
Application UI code in Tailwind CSS
</Timeline.Title>
<Timeline.Body>
Get access to over 20+ pages including a dashboard layout, charts, kanban board, calendar, and pre-order E-commerce & Marketing pages.
</Timeline.Body>
<Button color="gray">
Learn More
<ArrowSmallRightIcon className="ml-2 h-3 w-3" />
</Button>
</Timeline.Content>
</Timeline.Item>
<Timeline.Item>
<Timeline.Point />
<Timeline.Content>
<Timeline.Time>
March 2022
</Timeline.Time>
<Timeline.Title>
Marketing UI design in Figma
</Timeline.Title>
<Timeline.Body>
All of the pages and components are first designed in Figma and we keep a parity between the two versions even as we update the project.
</Timeline.Body>
</Timeline.Content>
</Timeline.Item>
<Timeline.Item>
<Timeline.Point />
<Timeline.Content>
<Timeline.Time>
April 2022
</Timeline.Time>
<Timeline.Title>
E-Commerce UI code in Tailwind CSS
</Timeline.Title>
<Timeline.Body>
Get started with dozens of web components and interactive elements built on top of Tailwind CSS.
</Timeline.Body>
</Timeline.Content>
</Timeline.Item>
</Timeline>
</div>
</div>
);
}
export default App;
結果
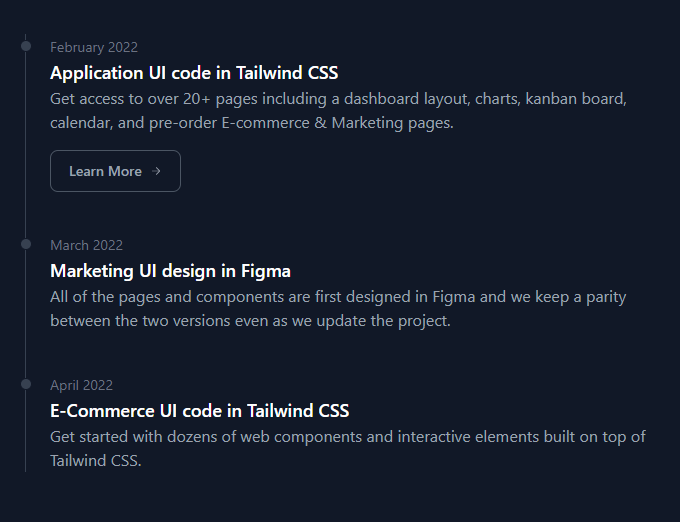
はい、できました。