[React+Tailwind] Flowbite で Navbar を表示してみる | 心を無にして始める React
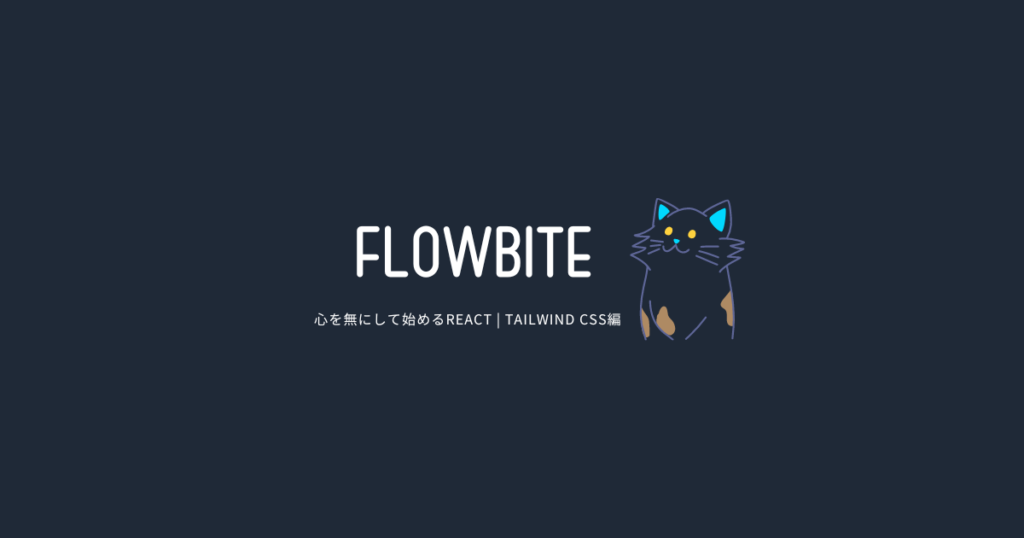
準備
Flowbite が使えるプロジェクトを準備します。
Navbar コンポーネントをつくる
./src/components 配下に Navbar.js を作ります。
import React, { forwardRef } from 'react';
import {
Navbar as FlowbiteNavbar,
} from 'flowbite-react';
const Navbar = forwardRef((
{
border = false,
children,
fluid = true,
menuOpen = false,
rounded = false,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar
border={border}
fluid={fluid}
menuOpen={menuOpen}
ref={ref}
rounded={rounded}
{...otherProps}
>
{children}
</FlowbiteNavbar>
);
});
const NavbarBrand = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Brand
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Brand>
);
});
const NavbarCollapse = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Collapse
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Collapse>
);
});
const NavbarLink = forwardRef((
{
active = false,
children,
disabled = false,
href,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Link
active={active}
disabled={disabled}
href={href}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Link>
);
});
const NavbarToogle = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteNavbar.Toggle
ref={ref}
{...otherProps}
>
{children}
</FlowbiteNavbar.Toggle>
);
});
export default Object.assign(Navbar, {
Brand: NavbarBrand,
Collapse: NavbarCollapse,
Link: NavbarLink,
Toggle: NavbarToogle,
});
Navbar コンポーネントをつかう
いつものように App.js を編集していきます。
import './App.css';
import Navbar from './components/Navbar';
function App() {
return (
<div className="min-h-screen flex flex-col dark:text-white dark:!bg-gray-900">
<Navbar
fluid={true}
rounded={false}
>
<Navbar.Brand href="https://neko-note.org/">
<img
src="https://placehold.jp/48/000000/ffffff/320x100.png?text=Site%20LOGO&css=%7B%22background-color%22%3A%22%20transparent%22%7D"
className="mr-3 h-6 sm:h-9"
alt="Site Logo"
/>
</Navbar.Brand>
<Navbar.Toggle />
<Navbar.Collapse>
<Navbar.Link
href="/navbars"
active={true}
>
Home
</Navbar.Link>
<Navbar.Link href="/navbars">
About
</Navbar.Link>
<Navbar.Link href="/navbars">
Services
</Navbar.Link>
<Navbar.Link href="/navbars">
Pricing
</Navbar.Link>
<Navbar.Link href="/navbars">
Contact
</Navbar.Link>
</Navbar.Collapse>
</Navbar>
<div className="flex flex-grow justify-center items-center">
<div>
your contents.
</div>
</div>
</div>
);
}
export default App;
結果
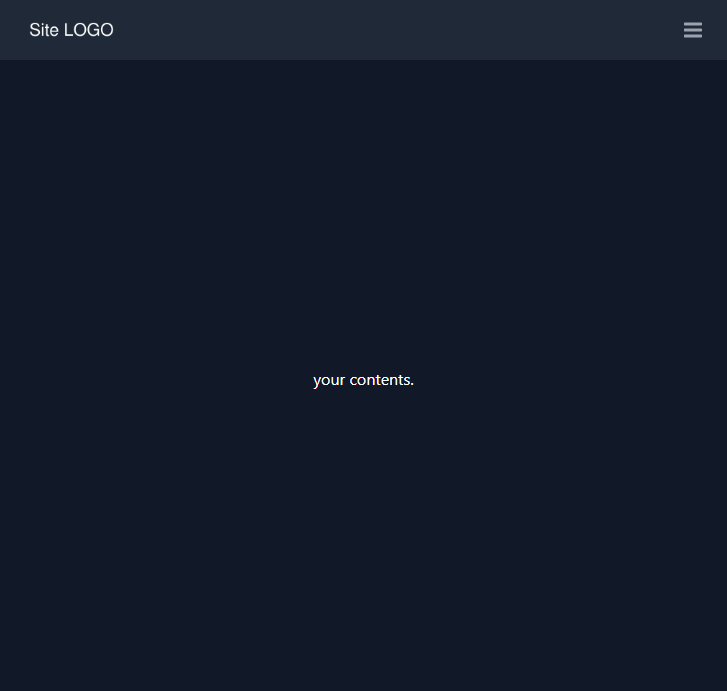
はい、できました。