[React] Axios で複数の通信を待ち合わせてみる | 心を無にして始める React
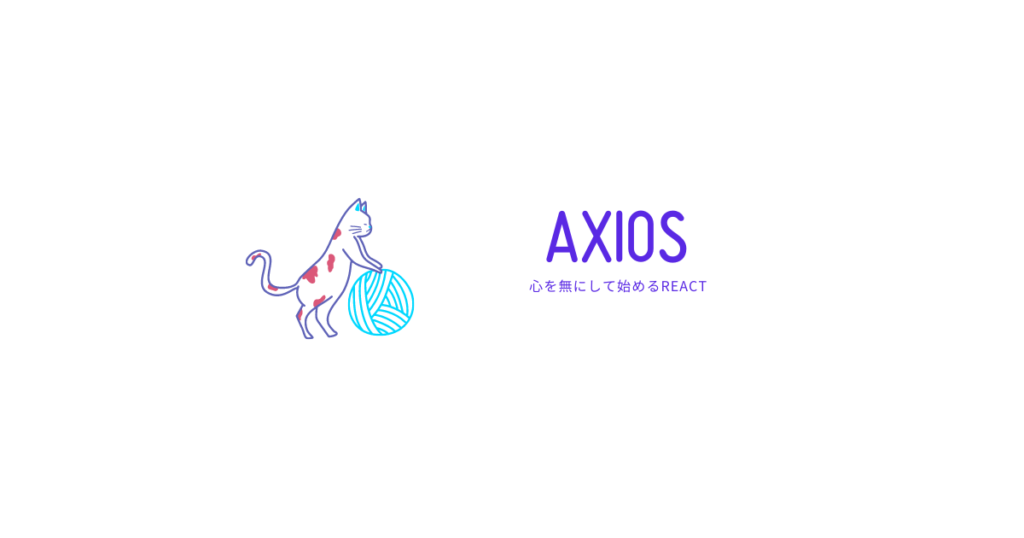
準備
Axios のインストールと使い方はこちら。
バックエンド
今回も json-server です。
使う json はこちら。
{
"cats": [
{
"id": 1,
"name": "Norwegian Forest Cat"
},
{
"id": 2,
"name": "Selkirk Rex"
},
{
"id": 3,
"name": "Munchkin"
}
],
"dogs": [
{
"id": 1,
"name": "といぷーどる"
},
{
"id": 2,
"name": "ちわわ"
},
{
"id": 3,
"name": "しば"
}
]
}
複数の通信を待ち合わせる
イメージ
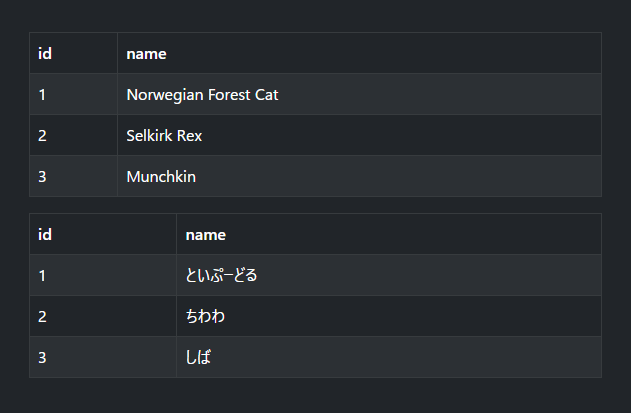
局所的なサンプル
Promise.all([
axios.get('/cats'),
axios.get('/dogs'),
]).then(([cats, dogs]) => {
// 全ての通信が完了したあとの処理
})
サンプルコード
それでは、例によって App.js を編集します。
import axios from 'axios';
import React, { useEffect, useState } from 'react';
import './App.css';
import Table from './components/Table';
axios.defaults.headers.get['Content-Type'] = 'application/json';
axios.defaults.headers.get.Accept = 'application/json';
axios.defaults.baseURL = 'http://localhost:3000/';
const COLUMNS = ['id', 'name'];
function App() {
const [pets, setPets] = useState({
cats: [],
dogs: [],
});
useEffect(() => {
Promise.all([
axios.get('/cats'),
axios.get('/dogs'),
]).then(([cats, dogs]) => {
setPets({
cats: cats.data,
dogs: dogs.data,
})
})
}, []);
return (
<>
<div className="bg-dark" style={{ minHeight: '100vh' }}>
<div className="p-5">
<Table
bordered
hover
striped
variant="dark"
columns={COLUMNS}
rows={pets?.cats?.map(pet => [pet.id, pet.name])}
/>
<Table
bordered
hover
striped
variant="dark"
columns={COLUMNS}
rows={pets?.dogs?.map(pet => [pet.id, pet.name])}
/>
</div>
</div>
</>
);
}
export default App;
はい、できました。
ここにコードがないコンポーネントは、過去の記事にあります ('◇’)ゞ