[React+Tailwind] Flowbite で Card を表示してみる | 心を無にして始める React
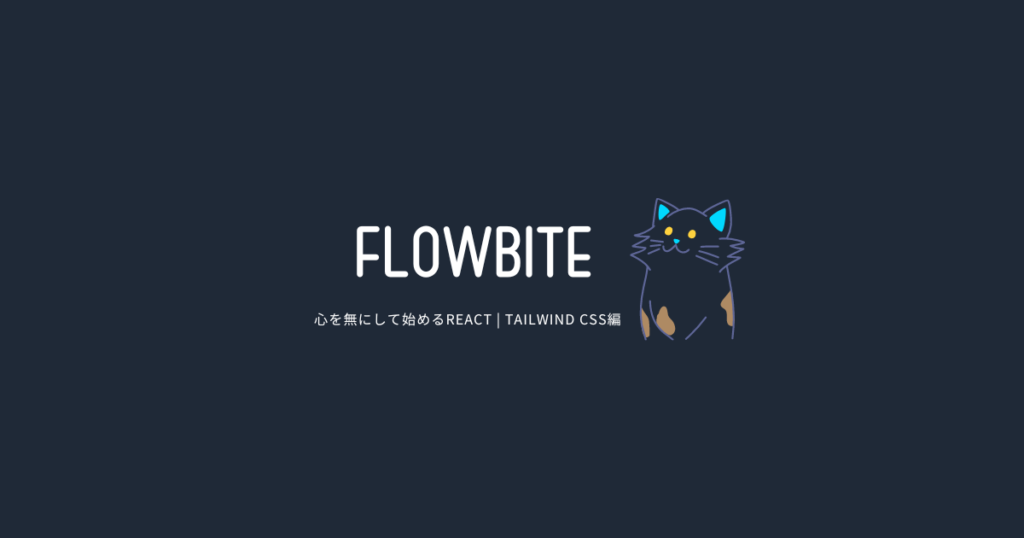
準備
Flowbite が使えるプロジェクトを準備します。
Card コンポーネントをつくる
./src/components 配下に Card.js を作ります。
import { forwardRef } from 'react';
import { Card as FlowbiteCard } from 'flowbite-react';
const Card = forwardRef((
{
children,
horizontal,
href,
imgAlt,
imgSrc,
...otherProps
},
ref,
) => {
return (
<FlowbiteCard
horizontal={horizontal}
href={href}
imgAlt={imgAlt}
imgSrc={imgSrc}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteCard>
);
});
export default Card;
Card コンポーネントをつかう
いつものように App.js を編集していきます。
import Card from './components/Card';
import './App.css';
function App() {
return (
<div className="dark">
<div className="h-screen p-8 flex flex-col justify-center items-center dark:!bg-gray-900">
<div className="flex gap-3">
<Card href="#">
<h5 className="text-2xl font-bold tracking-tight text-gray-900 dark:text-white">
Noteworthy technology acquisitions 2021
</h5>
<p className="font-normal text-gray-700 dark:text-gray-400">
Here are the biggest enterprise technology acquisitions of 2021 so far, in reverse chronological order.
</p>
</Card>
</div>
<div className="mt-4 flex gap-3">
<div className="max-w-sm">
<Card
imgAlt="Dummy image alt"
imgSrc="https://placehold.jp/16/3d4070/ffffff/256x256.png"
>
<h5 className="text-2xl font-bold tracking-tight text-gray-900 dark:text-white">
Noteworthy technology acquisitions 2021
</h5>
<p className="font-normal text-gray-700 dark:text-gray-400">
Here are the biggest enterprise technology acquisitions of 2021 so far, in reverse chronological order.
</p>
</Card>
</div>
<div className="max-w-sm">
<Card
horizontal={true}
imgAlt="Dummy image alt"
imgSrc="https://placehold.jp/16/3d4070/ffffff/256x256.png"
>
<h5 className="text-2xl font-bold tracking-tight text-gray-900 dark:text-white">
Noteworthy technology acquisitions 2021
</h5>
<p className="font-normal text-gray-700 dark:text-gray-400">
Here are the biggest enterprise technology acquisitions of 2021 so far, in reverse chronological order.
</p>
</Card>
</div>
</div>
</div>
</div>
);
}
export default App;
結果
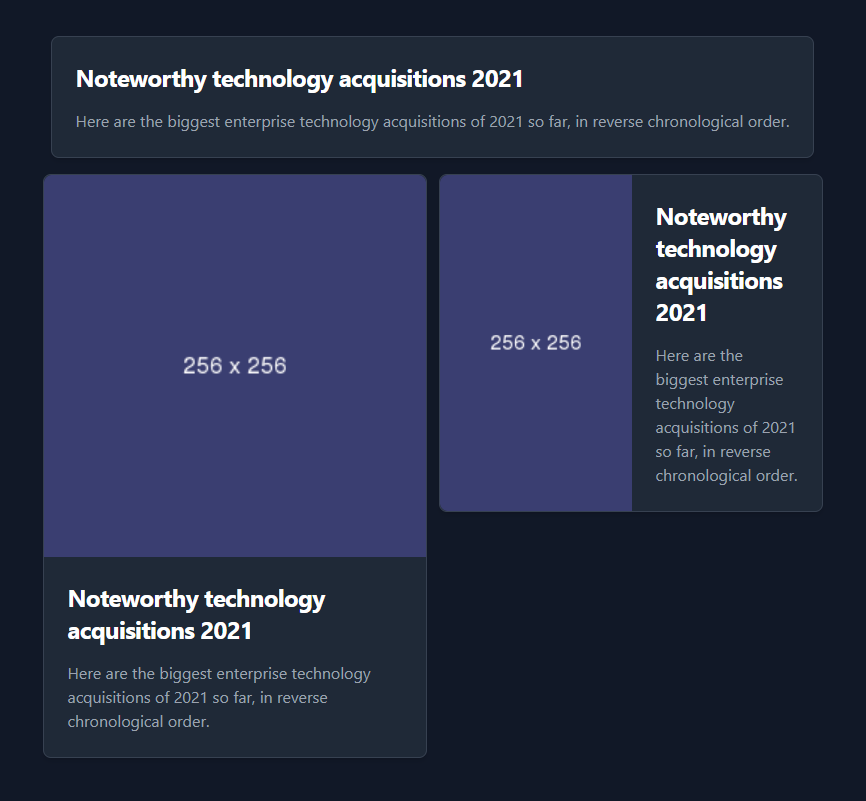
はい、できました。