[React+Tailwind] Flowbite で Table を表示してみる | 心を無にして始める React
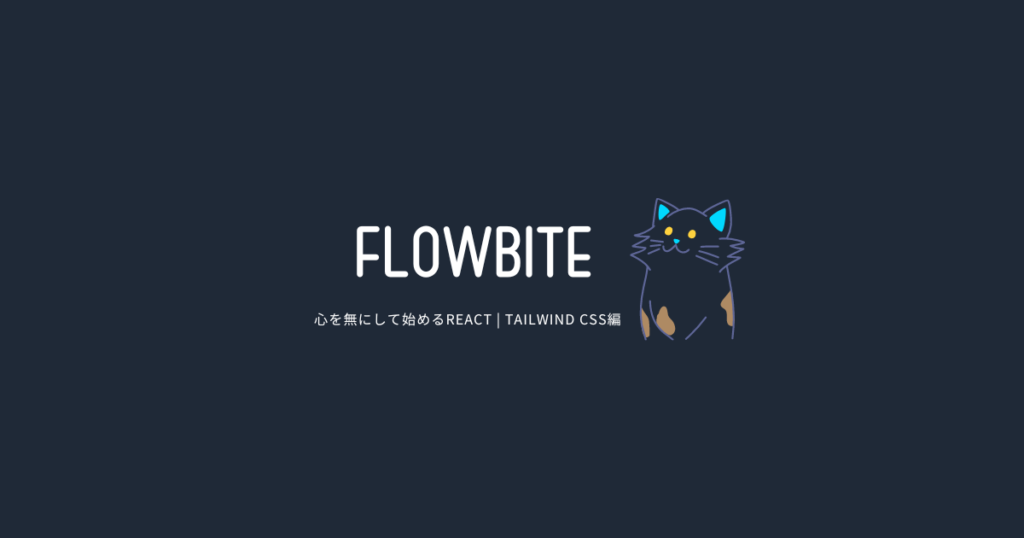
準備
Flowbite が使えるプロジェクトを準備します。
Table コンポーネントをつくる
./src/components 配下に Table.js を作ります。
import React, { forwardRef } from 'react';
import {
Table as FlowbiteTable,
} from 'flowbite-react';
const Table = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable>
);
});
const TableHead = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Head
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Head>
);
});
const TableHeadCell = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.HeadCell
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.HeadCell>
);
});
const TableBody = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Body
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Body>
);
});
const TableRow = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Row
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Row>
);
});
const TableCell = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Cell
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Cell>
);
});
export default Object.assign(Table, {
Head: TableHead,
HeadCell: TableHeadCell,
Body: TableBody,
Row: TableRow,
Cell: TableCell,
});
Table コンポーネントをつかう
いつものように App.js を編集していきます。
import './App.css';
import Form from './components/Form';
import Table from './components/Table';
function App() {
return (
<div className="min-h-screen w-full gap-4 flex flex-col justify-center items-center dark:text-white dark:!bg-gray-900">
<div className="w-full p-8">
<Table hoverable={true}>
<Table.Head>
<Table.HeadCell className="!p-4">
<Form.Checkbox />
</Table.HeadCell>
<Table.HeadCell>
Product name
</Table.HeadCell>
<Table.HeadCell>
Color
</Table.HeadCell>
<Table.HeadCell>
Category
</Table.HeadCell>
<Table.HeadCell>
Price
</Table.HeadCell>
<Table.HeadCell>
<span className="sr-only">
Edit
</span>
</Table.HeadCell>
</Table.Head>
<Table.Body className="divide-y">
<Table.Row className="bg-white dark:border-gray-700 dark:bg-gray-800">
<Table.Cell className="!p-4">
<Form.Checkbox />
</Table.Cell>
<Table.Cell className="whitespace-nowrap font-medium text-gray-900 dark:text-white">
Apple MacBook Pro 17"
</Table.Cell>
<Table.Cell>
Sliver
</Table.Cell>
<Table.Cell>
Laptop
</Table.Cell>
<Table.Cell>
$2999
</Table.Cell>
<Table.Cell>
<a
href="/tables"
className="font-medium text-blue-600 hover:underline dark:text-blue-500"
>
Edit
</a>
</Table.Cell>
</Table.Row>
<Table.Row className="bg-white dark:border-gray-700 dark:bg-gray-800">
<Table.Cell className="!p-4">
<Form.Checkbox />
</Table.Cell>
<Table.Cell className="whitespace-nowrap font-medium text-gray-900 dark:text-white">
Microsoft Surface Pro
</Table.Cell>
<Table.Cell>
White
</Table.Cell>
<Table.Cell>
Laptop PC
</Table.Cell>
<Table.Cell>
$1999
</Table.Cell>
<Table.Cell>
<a
href="/tables"
className="font-medium text-blue-600 hover:underline dark:text-blue-500"
>
Edit
</a>
</Table.Cell>
</Table.Row>
<Table.Row className="bg-white dark:border-gray-700 dark:bg-gray-800">
<Table.Cell className="!p-4">
<Form.Checkbox />
</Table.Cell>
<Table.Cell className="whitespace-nowrap font-medium text-gray-900 dark:text-white">
Magic Mouse 2
</Table.Cell>
<Table.Cell>
Black
</Table.Cell>
<Table.Cell>
Accessories
</Table.Cell>
<Table.Cell>
$99
</Table.Cell>
<Table.Cell>
<a
href="/tables"
className="font-medium text-blue-600 hover:underline dark:text-blue-500"
>
Edit
</a>
</Table.Cell>
</Table.Row>
</Table.Body>
</Table>
</div>
</div>
);
}
export default App;
結果
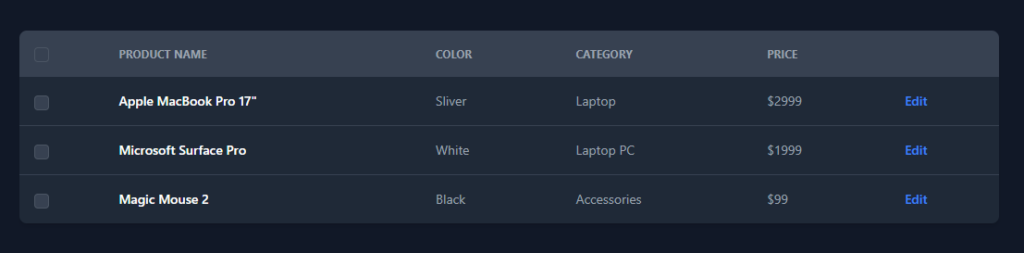
はい、できました。