[React] TanStack Table v8 を React で使ってみる | 心を無にして始める React
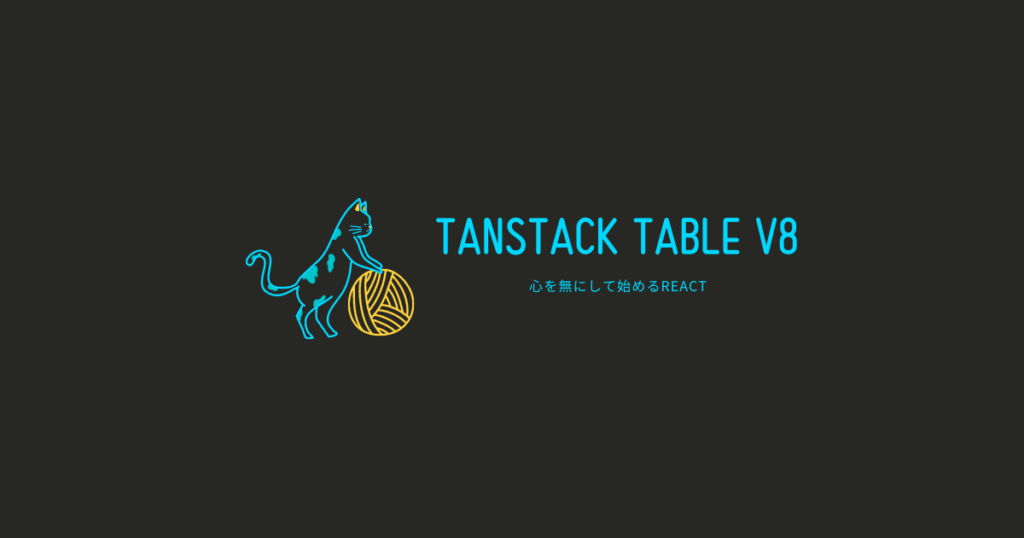
TanStask Table v8
準備
インストール
npm install @tanstack/react-table
react-table
イメージ
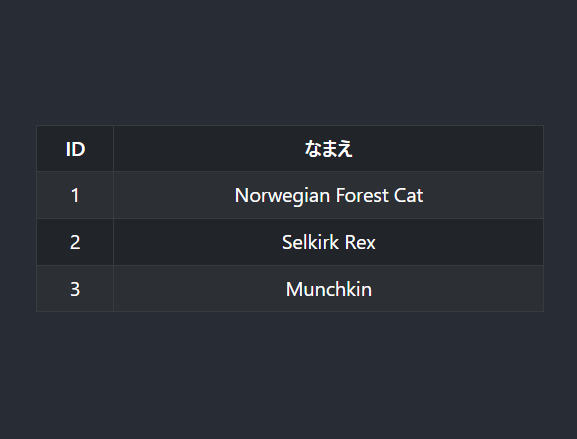
Table.js を編集する
ここで作った Table.js を編集していきます。
こんな感じです。
import React from 'react';
import { Table as BootstrapTable } from 'react-bootstrap';
import { useReactTable, flexRender, getCoreRowModel } from '@tanstack/react-table'
const Table = React.forwardRef(({
columns,
rows,
...otherProps
}, ref) => {
const table = useReactTable({
columns,
data: rows,
getCoreRowModel: getCoreRowModel(),
})
return (
<BootstrapTable ref={ref} {...otherProps}>
<thead>
{
table.getHeaderGroups().map(headerGroup => (
<tr key={headerGroup.id}>
{
headerGroup.headers.map(header => {
return (
<th key={header.id}>
{
header.isPlaceholder
? null
: flexRender(header.column.columnDef.header, header.getContext())
}
</th>
)
})
}
</tr>
))
}
</thead>
<tbody>
{
table.getRowModel().rows.map(row => (
<tr key={row.id}>
{
row.getVisibleCells().map(cell => (
<td key={cell.id}>
{flexRender(cell.column.columnDef.cell, cell.getContext())}
</td>
))
}
</tr>
))
}
</tbody>
</BootstrapTable>
)
})
export default Table;
使ってみる
では、いつものように App.js を編集していきます。
前回の記事の App.js をベースに進めます。
Axios で json-server と通信してデータを持ってきて、テーブルを表示します。
今後、テーブルとしての機能(ソート、フィルタ、ページネーションなど)を増やすときにも、あまり変わらない見た目で進めたいので、Bootstrap っぽい見た目はすこし維持しました (。´・ω・)
いつものように サンプルコード ぺたり (._.)
import axios from 'axios';
import { Suspense, useState } from 'react';
import { Spinner } from 'react-bootstrap';
import './App.css';
import Table from './components/Table';
axios.defaults.headers.get['Content-Type'] = 'application/json';
axios.defaults.headers.get.Accept = 'application/json';
axios.defaults.baseURL = 'http://localhost:3000/';
const COLUMNS = [
{
accessorKey: 'id',
header: 'ID',
},
{
accessorKey: 'name',
header: 'なまえ',
},
];
const Pets = ({
values,
setState,
}) => {
if (values === null) {
if (setState) {
throw axios.get('/cats').then(response => setState(response.data));
}
}
return (
<Table
bordered
hover
striped
variant="dark"
columns={COLUMNS}
rows={values}
/>
);
};
function App() {
const [cats, setCats] = useState(null);
return (
<div className="App">
<header className="App-header p-5">
<Suspense fallback={<Spinner animation="border" variant="light" />}>
<Pets values={cats} setState={setCats} />
</Suspense>
</header>
</div>
);
}
export default App;
はい、できました。