[React+Tailwind] Tableを TypeScript に書き直してみた | 心を無にして始める React
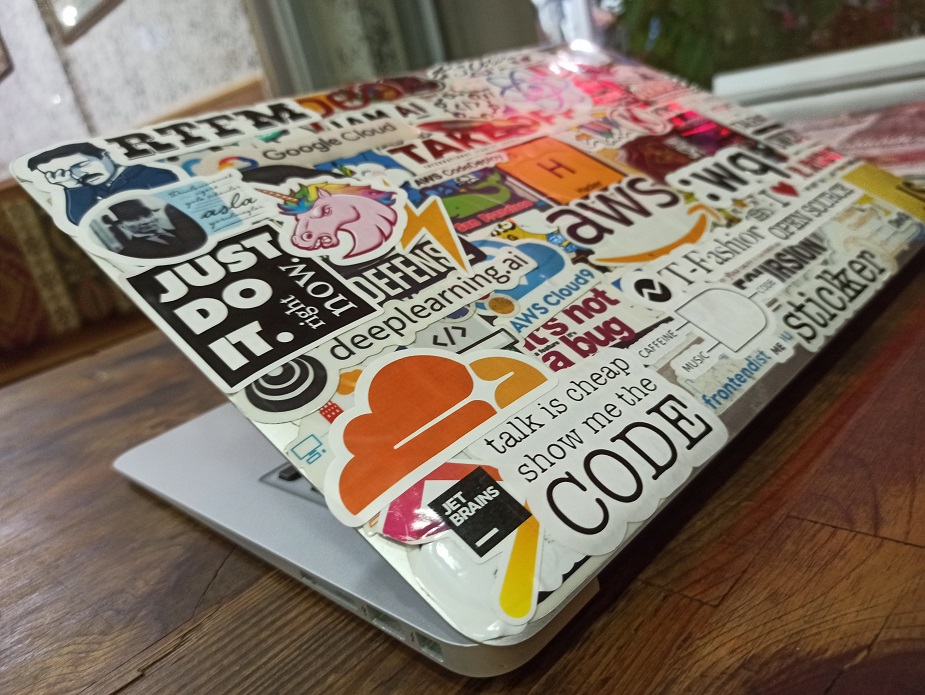
準備
書き直すベースはこちらの Table コンポーネントです。
JavaScript
ベースの Table.js です。
import React, { forwardRef } from 'react';
import {
Table as FlowbiteTable,
} from 'flowbite-react';
const Table = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable>
);
});
const TableHead = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Head
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Head>
);
});
const TableHeadCell = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.HeadCell
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.HeadCell>
);
});
const TableBody = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Body
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Body>
);
});
const TableRow = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Row
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Row>
);
});
const TableCell = forwardRef((
{
children,
...otherProps
},
ref,
) => {
return (
<FlowbiteTable.Cell
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Cell>
);
});
export default Object.assign(Table, {
Head: TableHead,
HeadCell: TableHeadCell,
Body: TableBody,
Row: TableRow,
Cell: TableCell,
});
TypeScript
Table.tsx として編集します。
import React, { ComponentProps, forwardRef, LegacyRef } from 'react';
import {
Table as FlowbiteTable,
TableProps as FlowbiteTableProps,
} from 'flowbite-react';
type TableProps = {} & FlowbiteTableProps;
const Table = forwardRef((
{
children,
...otherProps
}: TableProps,
ref: LegacyRef<HTMLTableElement>,
) => {
return (
<FlowbiteTable
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable>
);
});
type TableHeadProps = {} & ComponentProps<typeof FlowbiteTable.Head>;
const TableHead = forwardRef((
{
children,
...otherProps
}: TableHeadProps,
ref: LegacyRef<HTMLTableSectionElement>,
) => {
return (
<FlowbiteTable.Head
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Head>
);
});
type TableHeadCellProps = {} & ComponentProps<typeof FlowbiteTable.HeadCell>;
const TableHeadCell = forwardRef((
{
children,
...otherProps
}: TableHeadCellProps,
ref: LegacyRef<HTMLTableHeaderCellElement>,
) => {
return (
<FlowbiteTable.HeadCell
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.HeadCell>
);
});
type TableBodyProps = {} & ComponentProps<typeof FlowbiteTable.Body>;
const TableBody = forwardRef((
{
children,
...otherProps
}: TableBodyProps,
ref: LegacyRef<HTMLTableSectionElement>,
) => {
return (
<FlowbiteTable.Body
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Body>
);
});
type TableRowProps = {} & ComponentProps<typeof FlowbiteTable.Row>;
const TableRow = forwardRef((
{
children,
...otherProps
}: TableRowProps,
ref: LegacyRef<HTMLTableRowElement>,
) => {
return (
<FlowbiteTable.Row
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Row>
);
});
type TableCellProps = {} & ComponentProps<typeof FlowbiteTable.Cell>;
const TableCell = forwardRef((
{
children,
...otherProps
}: TableCellProps,
ref: LegacyRef<HTMLTableCellElement>,
) => {
return (
<FlowbiteTable.Cell
ref={ref}
{...otherProps}
>
{children}
</FlowbiteTable.Cell>
);
});
export default Object.assign(Table, {
Head: TableHead,
HeadCell: TableHeadCell,
Body: TableBody,
Row: TableRow,
Cell: TableCell,
});
結果
変化はないので、前と同じ。
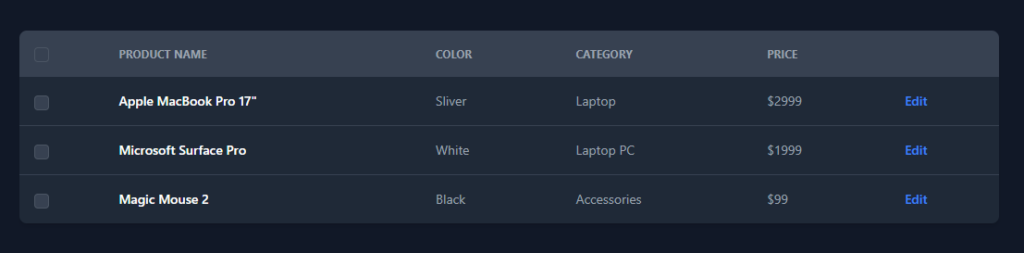
はい、できました。