[React+Tailwind] Flowbite で Rating を表示してみる | 心を無にして始める React
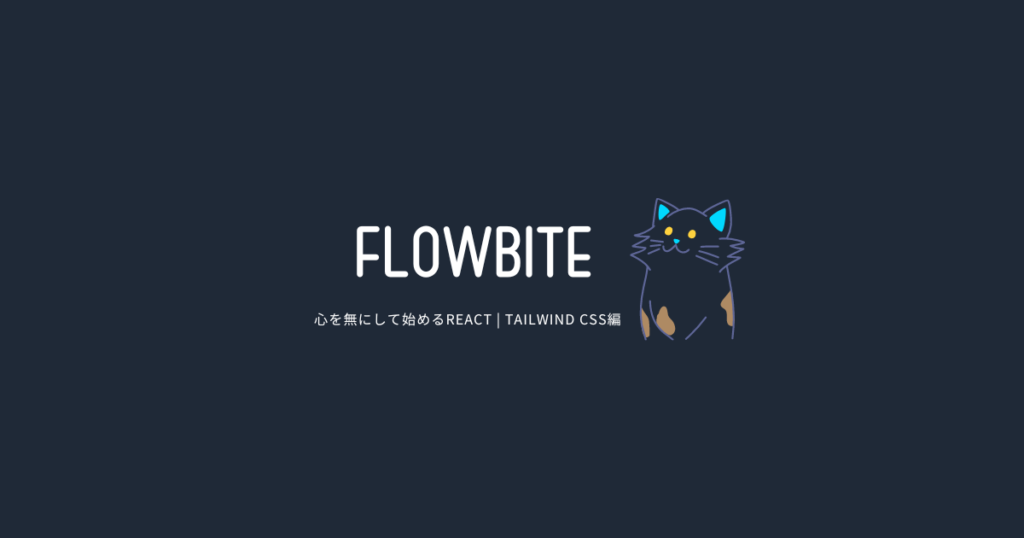
準備
Flowbite が使えるプロジェクトを準備します。
Rating コンポーネントをつくる
./src/components 配下に Rating.js を作ります。
import React, { forwardRef } from 'react';
import {
Rating as FlowbiteRating,
} from 'flowbite-react';
const Rating = forwardRef((
{
children,
size,
...otherProps
},
ref,
) => {
return (
<FlowbiteRating
ref={ref}
size={size}
{...otherProps}
>
{children}
</FlowbiteRating>
);
});
const RatingStar = (
{
filled = false,
starIcon,
...otherProps
}
) => {
return (
<FlowbiteRating.Star
filled={filled}
starIcon={starIcon}
{...otherProps}
/>
);
};
const RatingAdvanced = forwardRef((
{
children,
percentFilled,
...otherProps
},
ref,
) => {
return (
<FlowbiteRating.Advanced
percentFilled={percentFilled}
ref={ref}
{...otherProps}
>
{children}
</FlowbiteRating.Advanced>
);
});
export default Object.assign(Rating, {
Star: RatingStar,
Advanced: RatingAdvanced,
});
Rating コンポーネントをつかう
いつものように App.js を編集していきます。
import './App.css';
import Rating from './components/Rating';
function App() {
return (
<div className="min-h-screen flex justify-center items-center dark:text-white dark:!bg-gray-900">
<div className="flex flex-col gap-4">
<Rating>
<Rating.Star />
<Rating.Star />
<Rating.Star />
<Rating.Star />
<Rating.Star filled={false} />
<p className="ml-2 text-sm font-medium text-gray-500 dark:text-gray-400">
4.95 out of 5
</p>
</Rating>
<p className="text-sm font-medium text-gray-500 dark:text-gray-400">
1,745 global ratings
</p>
<Rating.Advanced percentFilled={70}>
5 star
</Rating.Advanced>
<Rating.Advanced percentFilled={17}>
4 star
</Rating.Advanced>
<Rating.Advanced percentFilled={8}>
3 star
</Rating.Advanced>
<Rating.Advanced percentFilled={4}>
2 star
</Rating.Advanced>
<Rating.Advanced percentFilled={1}>
1 star
</Rating.Advanced>
</div>
</div>
);
}
export default App;
結果
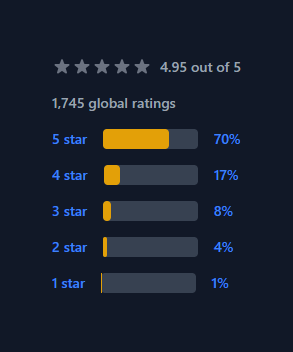
はい、できました。